How to Run PHP and MongoDB with Docker Compose and Apache
Posted November 18, 2023
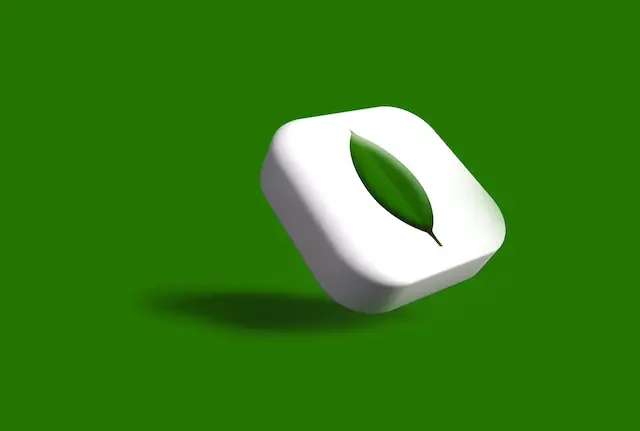
PHP runs great using a MongoDB database, especially in a Dockerized environment. In this guide, you’ll create a docker environment to set up a complete Docker Compose Apache PHP MongoDB app.
You will learn everything you need to get PHP up and running within Docker using Docker Compose, MongoDB, and Apache. You’ll cover the following topics:
- The best way to run a PHP app with MongoDB and Docker is to compose alongside the Apache server.
- How to install PHP MongoDB extensions using Docker php ext install MongoDB within Docker and use PHP to access them.
- Build a PHP app that shows you how to connect the PHP Apache server and MongoDB on Docker with CRUD operations.
- How to use Mongo Express to access MongoDB using GUI.
- How to use MongoDB Compass to manage a Dockerized PHP MongoDB instance.
Now, dive into this comprehensive and explore the world of Docker and Apache, PHP setup with Docker Compose, and MongoDB.
Related:
Step 1: Prerequisites
Creating a Docker Compose Apache PHP MongoDB environment is a step-by-step guide. You need to ensure you have:
- Docker installed on your computer
- Know how to work with PHP and MongoDB.
- Based on this guide, you will use the following application structure throughout the article.
php-docker/
│ ├── Dockerfile
│ └── docker-compose.yml
└── app/
└── index.php
Before setting up PHP and MongoDB with Docker, ensure you have the above files ready. Let’s break it down:
This structure will contain all the Docker Apache PHP and MongoDB settings you need as follows:
Dockerfile
contains all the instructions to package a PHP image.docker-compose.yml
defines your Docker Compose services for your app. These services will be Mongo Express, MongoDB, and PHP with Apache.app/index.php
hosts your PHP app source code.
Step 2: Creating PHP Apache Server Image using PHP Dockerfile
You need to build a custom PHP image that Docker Compose will use to spin up your app. This will let you choose the version of PHP you want to run and install the extensions you need.
Dockerfile
allows you to do all this based on your app. Dockerfile
adds the configurations required to package your application together. Go ahead and create a Dockerfile
, add a PHP Docker base image with Apache environment, and install the PHP MongoDB extension as follows:
- Use PHP Apache as your base image as follows:
# Use an official PHP Apache runtime
FROM php:8.2-apache
# Enable Apache modules
RUN a2enmod rewrite
- This Dockerfile will download PHP
8.2
as your base image. If you need to use a different version, change8.2
, and everything will still work fine. - Use
RUN
to enable PHP Apache modules
# Install MongoDB extension
RUN pecl install mongodb \
&& docker-php-ext-enable mongodb
- The command
pecl install mongodb
will install the MongoDB extension, anddocker-php-ext-enable mongodb
will enable it within Docker.
This Dockerfile is enough to spin up Apache PHP MongoDB. It should be complete as follows:
# The official PHP Apache runtime
FROM php:8.2-apache
# Enable Apache modules
RUN a2enmod rewrite
# Install MongoDB extension and enable it within Docker
RUN pecl install mongodb \
&& docker-php-ext-enable mongodb
Step 3: Creating PHP Apache Container with Docker Compose and Serve PHP App
A container will run the Dockerfile you have created. However, you need Docker Compose to package PHP.
Head over to your docker-compose.yml
file and create PHP Apache and MongoDB service as follows:
version: '3.9'
services:
# PHP Apache service configuration
php-apache:
build:
# The Dockerfile path
context: .
dockerfile: Dockerfile
# Mount the local ./app directory to /var/www/html in the container
volumes:
- ./app:/var/www/html
# Expose port 8000 on the host and map it to port 80 in the container
ports:
- 8000:80
# Define a dependency on the mongodb service
depends_on:
- mongodb
# MongoDB service configuration
mongodb:
image: mongo:latest
# Expose MongoDB default port
ports:
- "27017:27017"
php-apache
packages your PHP source code withinapp/index.php
and uses Apache to expose it to the web.- A
mongodb
service will be created and exposed to MongoDB on Docker on port 27017. - Make sure you have
depends_on
in your compse. It tells thePHP-apache
container to use themongodb
service. This way. The MongoDB must be up and running so PHP can access it.
Step 4: Adding MongoDB Connection to PHP Apache using Docker Compose
Your goal is to ensure PHP can access MongoDB and create Database Connections. Go to the app/index.php
file and add MongoDB PHP connection as follows:
<?php
$mongoHost = 'mongodb'; // The hostname of the MongoDB server (the service name in Docker Compose)
$mongoPort = 27017; // The port on which MongoDB is running
try {
// Attempt to create a new MongoDB client
$mongoClient = new MongoDB\Driver\Manager("mongodb://{$mongoHost}:{$mongoPort}");
// If successful, print a success message
echo "Connected to MongoDB successfully!";
} catch (Exception $e) {
// If an exception occurs, print an error message with the details
echo "Failed to connect to MongoDB: " . $e->getMessage();
}
?>
The key things you need to note here are:
- The Dockerized PHP app will connect to MongoDB using the URL
mongodb://mongodb:27017"
. - At the same time,
mongoHost
equalsmongodb
. This must be the name of the service you use to run MongoDB. This URI must reflect as such. Otherwise, your PHP script will fail to connect to the database.
Rerun Docker Compose to build your containers:
docker-compose up -d --build
If you open http://localhost:8000/
on your browser, Apache will execute the PHP script in your index.php
file and connect to the MongoDB database. If successful, you should have a database connection:
Step 5: Accessing MongoDB PHP Apache Server using Mongo Express
You can now use your Docker Compose Apache PHP, and MongoDB set up in a Dockerized environment. However, you need a way to access your database.
On the local machine, MongoDB uses Compass as your UI. But when running on Docker, you need to use Mongo Express to access what’s happening in your DB:
Go ahead and update the docker-compose.yml
file with Mongo Express as follows:
version: '3.9'
services:
# PHP Apache service
php-apache:
build:
# the Dockerfile path
context: .
dockerfile: Dockerfile
# Mount the local ./app directory to /var/www/html in the container
volumes:
- ./app:/var/www/html
ports:
- 8000:80
# Define a dependency on the mongodb service
depends_on:
- mongodb
# MongoDB service
mongodb:
image: mongo:latest
container_name: mongodb
ports:
- "27017:27017"
environment:
# MongoDB authentication credentials
MONGO_INITDB_ROOT_USERNAME: admin
MONGO_INITDB_ROOT_PASSWORD: pass
volumes:
# Mount local directories for MongoDB data persistence
- ./db_data/:/data/db/
- mongodb-data:/data/db
networks:
- mynetwork
# MongoDB Express service
mongo-express:
image: mongo-express:latest
container_name: mongo-express
ports:
- "8081:8081"
environment:
# MongoDB Express configuration
ME_CONFIG_MONGODB_ADMINUSERNAME: admin
ME_CONFIG_MONGODB_ADMINPASSWORD: pass
ME_CONFIG_MONGODB_URL: mongodb://admin:pass@mongodb:27017/
networks:
- mynetwork
# volumes for MongoDB data persistence
volumes:
mongodb-data:
db_data:
# custom network for communication between services
networks:
mynetwork:
The database connection URI will change from mongodb://mongodb:27017
to mongodb://admin:pass@mongodb:27017/
when using the above update. You have added a user and password to access MongoDB. This must be updated to your MongoDB Connection URI with mongodb://your_username:your_password@mongodb:27017/
Step 6: Using Mongo Express with Docker and PHP
At this point, your app/index.php
code must be updated to match the new URI. You can go further and add more code to add data and fetch from MongoDB as follows:
<?php
try {
$mongoClient = new MongoDB\Driver\Manager("mongodb://admin:pass@mongodb:27017/");
echo "Connected to MongoDB successfully!";
} catch (Exception $e) {
echo "Failed to connect to MongoDB: " . $e->getMessage();
}
Go ahead and rerun Docker Compose to rebuild and run your containers:
docker-compose up -d --build
Open http://localhost:8081/
to access Mongo Express.
Then use Username admin
and password as pass
to log in:
Conclusion
This guide taught you how to use PHP with MongoDB and Docker Compose alongside Apache server. Use this setup to build your Dockerized PHP apps with MongoDB and access the DB using Mongo Express. I hope you found this guide helpful!
Happy Coding!