Easy Typeorm MariaDB Example Guide with Nest.js
Posted January 27, 2024
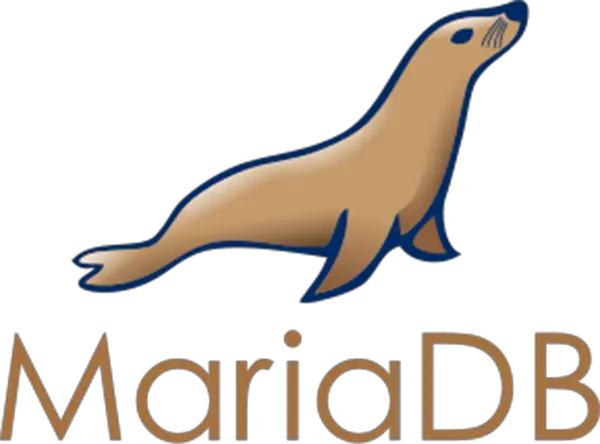
TypeORM is dive. It works so well when used alongside Nest.js. This way, you can interact with databases such as MariaDB. MariaDB is an SQL database. Now, TypeORM will allow the use of ORM models within your Nest.js code. This way, TypeORM will take over MariaDB and ensure your databases are updated.
Here, you do not need to use any SQL-related scripts. TypeORM will use code to model and mimic your tables with code. Ason Note. Nest.js and TypeORM suport for MariaDB is same to that of MySQL. You only need to change connections to MariaDB if you have an existing MySQL TypeORM Nest.js app.
Ready? Dive into this easy guide and learn how to get TypeORM ready within Nest.js and exploit MariaDB capabilities.
What you Need to Run MariaDB with TypeORM and Nest.js
Before diving into this easy Typeorm MariaDB Guide with Nest.js, you need to ensure the following:
- Node.js is ready on your machine.
- You have prior knowledge working with Node.js or Nest.js.
- MariaDB must be installed and running on your computer.
Creating Nest.js App with TypeORM and MariaDB
You first need a working Nest.js and install TypeORM and MariaDB dependencies in these few steps:
- Install Nest.js CLI and use it to create a Nest.js App
# Install the CLI
npm i -g @nestjs/cli
# create the app
nest new nest-mariadb
- Change the directory and point to the newly created folder
cd nest-mariadb
- Use the following command to install TypeORM and MariaDB
npm install --save @nestjs/typeorm typeorm mariadb
Go ahead and create a module/resource based on the application you want to create:
nest g resource products --no-spec
I will create a REST API using Product as the featured module.
Adding TypeORM MariaDB Entity
An Entity represents database tables. An entity should correspond to a logic object to model and interact with the data stored in a database.
This means Entities will make it easier for TypeORM to work with the MariaDB database in an object-oriented way. You interact with data using familiar class-based structures rather than raw SQL.
To create your Entity, navigate to the src/products/product.entity.ts
file. Here, create a Product blueprint using TypeORM as follows:
// product.entity.ts
import { Entity, Column, PrimaryGeneratedColumn } from 'typeorm';
@Entity()
export class Product {
@PrimaryGeneratedColumn()
id: number;
@Column()
name: string;
@Column()
description: string;
@Column({ type: 'decimal', precision: 10, scale: 2 })
price: number;
// Additional properties based on your requirements
}
To use this Entity, you will need the feature product module to include the Product entity. Go to src/products/product.module.ts
and import your Entities and TypeORM:
import { TypeOrmModule } from '@nestjs/typeorm';
import { Product } from './product.entity';
Now, include the TypeOrmModule.forFeature([Product])
in the imports array:
imports: [TypeOrmModule.forFeature([Product])],
Connecting MariaDB with TypeORM and Nest.js
The next major change is to add database connections related to MariaDB. These changes must be added to the entry point of your Nest.js app. This means the changes will be added on app.module.ts
as follows:
imports: [
TypeOrmModule.forRoot({
// TypeORM configuration options (can be in a separate file, e.g., ormconfig.js)
type: 'mariadb',
host: 'localhost',
port: 3306,
username: 'your_db_username',
password: 'your_db_password',
database: 'your_db_name',
entities: [__dirname + '/**/*.entity{.ts,.js}'],
synchronize: true,
}),
Remember: MariaDB TypeORM connection is the same as that of MySQL. They use the same PORT. However, you only need to change
type
tomariadb
, and TypeORM will work fine.
Building CRUD App with Nest.js MariaDB and TypeORM
For this step, I will refer you to this Guide to TypeORM with NestJS, Postgres, MySQL. It covers how to use MySQL with TypeORM.
Essentially, MariaDB will use the same steps. You only need to check type: 'mariadb',
.
If you want the complete code sample, check out these GitHub repositories and adjust your connection code to maTCH MariaDB:
Conclusion
That’s all you need to know: Typeorm with MariaDB and Nest.js. I hope you can now use TypeORM and, create secure and easy connections to MariaDB, and access the whole server with Nest.js.